Thursday 24 November 2016
Wednesday 23 November 2016
Trace & Transpose of the Given Matrix(2-D Array C-Programs)
* Write a Program to fill 3 * 3 integer array with user input.
#include<stdio.h> #include<conio.h> void main() { int a[3][3],i,j; clrscr(); printf("Enter 3 * 3 Matrix:\n"); for(i=0;i<3;i++) { for(j=0;j<3;j++) { scanf("%d",&a[i][j]); } } printf("The Matrix values are:\n"); for(i=0;i<3;i++) { for(j=0;j<3;j++) { printf("%d ",a[i][j]); } printf("\n"); } getch(); } |
Output:-
* Write a Program input 3 * 3 integer array and print the trace of the give matrix.
#include<stdio.h>
#include<conio.h>
void main()
{
int a[3][3],trace=0,i,j;
clrscr();
printf("Enter 3 * 3 Matrix:\n");
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
scanf("%d",&a[i][j]);
}
}
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
if(i==j)
trace=trace+a[i][j];
}
}
printf("The Trace value is:%d",trace);
getch();
}
|
Output:-
* Write a Program input 3 * 3 integer array and print the transpose of the give matrix.
#include<stdio.h> #include<conio.h> void main() { int a[3][3],b[3][3],i,j; clrscr(); printf("Enter 3 * 3 Matrix:\n"); for(i=0;i<3;i++) { for(j=0;j<3;j++) { scanf("%d",&a[i][j]); } } for(i=0;i<3;i++) { for(j=0;j<3;j++) { b[i][j]=a[j][i]; } } printf("The Transpose Matrix values are:\n"); for(i=0;i<3;i++) { for(j=0;j<3;j++) { printf("%d ",b[i][j]); } printf("\n"); } getch(); } |
Output:-
________________________________________
_____________________________________________________________________________
C-Programs Using 2-D Array (Dynamic Time)
Dynamic time :-
By using this scanf() we can assining the values to dynamically ( or ) in runtime .In again we can assign the values in two ways using scanf().
1. Without using loops.
2. With using loops.
1. Without using loops :-
scanf("%d",& a[0][0]);
scanf("%d",&a[0][1]);
scanf("%d",& a[0][2]);
scanf("%d",&a[1][0]);
scanf("%d",& a[1][1]);
scanf("%d",& a[1][2]);
2. With using loops :-
for(i=1;i<=r-1;i++)
{
for(j=1;j<=c-1;j++)
scanf("%d",&a[i][j]);
}
Displaying 2 D values :-
for(i=1;i<=r-1;i++)
{
for(j=1;j<=c-1;j++)
printff("%d",a[i][j]);
printf("\n");
}
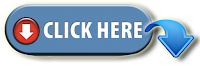
________________________________________
_____________________________________________________________________________
Monday 21 November 2016
Matrix C-Programs Using 2-D Array(4 Formats)
* Write a Program to fill 3 X 3 integer array with the fallowing format.
#include<stdio.h> #include<conio.h> void main() { int a[3][3],i,j; clrscr(); for(i=0;i<3;i++) { for(j=0;j<3;j++) a[i][j]=0; } for(i=0;i<3;i++) { for(j=0;j<3;j++) printf("%d ",a[i][j]); printf("\n"); } getch(); } |
* Write a Program to fill 3 X 3 integer array with the fallowing format.
#include<stdio.h> #include<conio.h> void main() { int a[3][3],i,j; clrscr(); for(i=0;i<3;i++) { for(j=0;j<3;j++) { if(i==j) a[i][j]=1; else a[i][j]=0; } } for(i=0;i<3;i++) { for(j=0;j<3;j++) printf("%d ",a[i][j]); printf("\n"); } getch(); } |
* Write a Program to fill 3 X 3 integer array with the fallowing format.
#include<stdio.h> #include<conio.h> void main() { int a[3][3],i,j; clrscr(); for(i=0;i<3;i++) { for(j=0;j<3;j++) { if(i>j) a[i][j]=3; else if(i==j) a[i][j]=1; else a[i][j]=2; } } for(i=0;i<3;i++) { for(j=0;j<3;j++) printf("%3d",a[i][j]); printf("\n"); } getch(); } |
* Write a Program to fill 5 X 5 integer array with the fallowing format.
#include<stdio.h> #include<conio.h> void main() { int a[5][5],i,j; clrscr(); for(i=0;i<5;i++) { for(j=0;j<5;j++) { if(i==0||j==0||i==4||j==4) a[i][j]=1; else if(i==2&&j==2) a[i][j]=5; else a[i][j]=0; } } for(i=0;i<5;i++) { for(j=0;j<5;j++) printf("%d ",a[i][j]); printf("\n"); } getch(); } |
________________________________________
_____________________________________________________________________________
Sunday 20 November 2016
Saturday 19 November 2016
C-Programs Using 2-D Array- (Static Time)(www.computersadda.com)
* In this above Format of 3 Ways to fill 3 X 3 integer array with user input.
With out using loops
#include<stdio.h> #include<conio.h> void main() { int a[3][3]={{4,5,6},{8,9,2},{2,7,9},}; clrscr(); printf("3 * 3 Matrix values are:\n"); printf("%d ",a[0][0]); printf("%d ",a[0][1]); printf("%d ",a[0][2]); printf("\n%d ",a[1][0]); printf("%d ",a[1][1]); printf("%d ",a[1][2]); printf("\n%d ",a[2][0]); printf("%d ",a[2][1]); printf("%d ",a[2][2]); getch(); } |
With using for loop
#include<stdio.h> #include<conio.h> void main() { int i,a[3][3]={{4,5,6},{8,9,2},{2,7,9},}; clrscr(); printf("3 * 3 Matrix values:\n"); for(i=0;i<3;i++) { printf("%d ",a[0][i]); } printf("\n"); for(i=0;i<3;i++) { printf("%d ",a[1][i]); } printf("\n"); for(i=0;i<3;i++) { printf("%d ",a[2][i]); } getch(); } |
With using nested for loop
#include<stdio.h> #include<conio.h> void main() { int i,j,a[3][3]={{4,5,6},{8,9,2},{2,7,9},}; clrscr(); printf("3 * 3 matrix values:\n"); for(i=0;i<3;i++) { for(j=0;j<3;j++) { printf("%d ",a[i][j]); } printf("\n"); } getch(); } |
________________________________________
_______________________________________________________________________________
Friday 18 November 2016
Thursday 17 November 2016
Quiz of the Computer Fundamentals (Week-20)
This set contains 10 multiple choice questions from Computer Fundamentals. Each question has four possible options and there is one and only one correct answer for each question.
Note:- explanation in below the questions
_________________________________________________________________________________Note:- explanation in below the questions
1. CD-ROM stands for
a. Compactable Read Only Memoryb. Compact Data Read Only Memory
c. Compactable Disk Read Only Memory
d. Compact Disk Read Only Memory
2. UNIVAC is
a. Universal Automatic Computer
b. Universal Array Computer
c. Unique Automatic Computer
d. Unvalued Automatic Computer
3. MSI stands for
a. Medium Scale Integrated Circuitsb. Medium System Integrated Circuits
c. Medium Scale Intelligent Circuit
d. Medium System Intelligent Circuit
4. The capacity of 3.5 inch floppy disk is
a. 1.40 MB
b. 1.44 GB
c. 1.40 GB
d. 1.44 MB
5. WAN stands for
a. Wire Area Network
b. Wide Area Network
c. Wide Array Net
d. Wireless Area Network
6. ALU is
a. Arithmetic Logic Unit
b. Array Logic Unit
c. Application Logic Unit
d. None of above
7. MICR stands for
a. Magnetic Ink Character Reader
b. Magnetic Ink Code Reader
c. Magnetic Ink Cases Reader
d. None
8. MU stands for
a. Memory Unit
b. Magnetic Memory
c. Main Memory
d. None
9. ASCII stands for
a. American Stable Code for International Interchange
b. American Standard Case for Institutional Interchange
c. American Standard Code for Information Interchange
d. American Standard Code for Interchange Information
10. EEPROM stand for
a. Electrically Erasable Programmable Read Only Memory
b. Easily Erasable Programmable Read Only Memory
c. Electronic Erasable Programmable Read Only Memory
d. None of the above
----------------------------------------------------------------------------------------------------------------------
Explanation
1.CD-ROM
There are no objects with the name as in other options. CD-ROM is a non-volatile optical data storage medium using the same physical format as audio compact disk, readable by a computer with a CD-ROM drive.
2. UNIVAC
There are no computers with the name as in other options. UNIVAC was the first general purpose electronic digital computer designed for commercial use, produced by Universal Accounting Company of John Mauchly and J.P.Eckert in 1951.
3. MSI
After the invention of IC chips the development of computers plunged into next phase. Small Scale Integration and Medium Scale Integration (SSI and MSI) were used in third generation of computers and Large Scale Integration and Very Large Scale Integration (LSI and VLSI) are being used in fourth generation of computers.
4. The capacity of 3.5 inch floppy disk is
Microfloppy disks (3.5 inch) if it is high density (MF2HD) can store 1.44 MB and if it is low density (MF2DD), it can store 720 KB. Mini Floppy disks (5.25 inch) if it is high density (MD2HD) can store 1.2 MB and low density (MD2DD) stores 360 KB of data.
5. WAN
There are three different classes of computer network namely, Local Area Network (LAN) that covers a small geographical area such as a room, a building or a compound; Metropolitan Area Network (MAN) that has a citywide coverage; and Wide Area Network (WAN) that covers the whole globe or beyond the globe.
6. ALU
ALU is a unit in Central Processing Unit in a computer system that is responsible for arithmetic calculations and logical operations. Apart from ALU, the CPU contains MU (Memory Unit) and CU (Control Unit).
7. MICR
MICR (Magnetic Ink Character Reader) is kind of scanner that can scan and identify the writing of magnetic ink. This device is used in banks to verify signatures in Checks.
8.MU
It is the location to store the information. It can be divided into 2 parts.
They are
A.Primary memory unit
B. secondary memory unit
9. ASCII
ASCII is a code which converts characters – letters, digits, punctuations and control characters such as Alt, Tab etc – into numeral form. ASCII code is used to represent data internally in micro-computers. ASCII codes are 7 bits and can represent 0 to 127 and extended ASCII are 8 bits that represents 0 to 255.
10. EEPROM
There are three types of ROM namely, PROM, EPROM and EEPROM. PROM can’t be reprogrammed, EPROM can be erased by exposing it in high intensity ultraviolet light and EEPROM can be erased and reprogrammed electrically. It is not needed to be removed from the computer to be modified.
---------------------------------------------------------------------------------------------------------------
---------------------------------------------------------------------------------------------------------------
Two Dimensional Array in C-Language
Multi dimensional Arrays :-
Collection of values with similar datatype using more than one subscription is known as a Multi dimensional Array.
'C' allows us to define tables of items by using two dimensional arrays.
Collection of values with similar datatype using two subscripts.It is also called table (or) Matrix.
Two dimensional arrays are declared as fallows
Syntax:-
data type array name[row size][column size];
int a[3][4];
We can create two types of rows & columns formats.
Format 1:-
Ex:- int a[3][4]
Format 2:-
Ex:- int a[3][4]
Assigning the values to 2D :-
We can Assign the values to 2D in two ways.
Those are
1. Static time
2. Dynamic time
1. Static time :-
In static time also we can assign the values in two ways.
1. 2D initialization
2. 2D assignment
1. 2D initialization :-
Assigning the values to 2D at the time 2D declaration.
Ex 1:-
int a[2][3]={{1,2,3},{4,5,6}};
Ex 2:-
int a[3][3]={{1,2,3},{4,5,6},{7,8,9}};
2. 2D assignment :-
Assigning the values to 2D after the variable declaration.
Ex:-
int a[2][3];
a[0][0]=1;
a[0][1]=2;
a[0][2]=3;
a[1][0]=4;
a[1][1]=5;
a[1][2]=6;
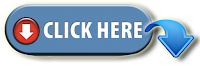
________________________________________
_______________________________________________________________________________
Subscribe to:
Posts
(
Atom
)